



Iniezione di modelli lato server (SSTI)
Quando esiste una vulnerabilità SSTI, i dati forniti dall'utente vengono iniettati in un modello senza un'adeguata sanificazione, consentendo agli aggressori di inserire payload dannosi. Ciò può portare all'esecuzione di codice arbitrario sul server, con potenziali gravi violazioni della sicurezza.
Scenari comuni in cui si verifica la SSTI
- Rendering dei contenuti generati dagli utenti senza un'adeguata sanificazione:
Molte applicazioni Web consentono agli utenti di creare o modificare contenuti, come profili, commenti o post. Se questo contenuto generato dall'utente viene renderizzato utilizzando un modello lato server senza un'adeguata sanificazione degli input, può portare a SSTI. Ad esempio, se il commento di un utente è incorporato direttamente in un modello, qualsiasi codice dannoso all'interno del commento può essere eseguito dal server.
- Utilizzo di modelli per la generazione di e-mail in base agli input dell'utente:
I modelli vengono spesso utilizzati per generare e-mail dinamiche, come e-mail di conferma, newsletter o reimpostazione della password. Se il contenuto di queste e-mail include input utente non sanificati, può causare SSTI. Ad esempio, se l'indirizzo o il nome e-mail di un utente è incluso nel modello di email senza sanificazione, un utente malintenzionato potrebbe inserire un codice dannoso, che porta all'esecuzione di questo codice sul server al momento della generazione dell'email.
- Generazione dinamica di report in cui gli utenti possono influenzare l'output:
Alcune applicazioni generano report dinamici in base agli input degli utenti. Questi report possono includere vari punti dati, filtri o opzioni di formattazione specificati dall'utente. Se questi input dell'utente sono integrati nel modello di report senza un'adeguata sanificazione, può portare a SSTI. Un utente malintenzionato potrebbe manipolare l'input per includere codice dannoso, che il server eseguirebbe durante la generazione del report.
Come funziona SSTI
Meccanismo alla base dei motori di template
I motori di template consentono agli sviluppatori di utilizzare modelli con segnaposto per contenuti dinamici. Questi segnaposto sono indicatori speciali all'interno del modello che vengono sostituiti con dati effettivi in fase di esecuzione. Lo scopo dell'utilizzo dei motori di template è separare la logica dell'elaborazione dei dati dal livello di presentazione, semplificando la gestione e la manutenzione del codice. Ad esempio, in un'applicazione Web, un modello può contenere segnaposto per il nome dell'utente, l'immagine del profilo e altri dettagli personali. Quando un utente accede al proprio profilo, il motore dei modelli sostituisce questi segnaposti con i dati corrispondenti dell'account dell'utente.
Come gli aggressori sfruttano l'SSTI
Gli aggressori sfruttano SSTI iniettando codice dannoso nei segnaposto all'interno dei modelli. Ciò si verifica in genere quando l'input dell'utente viene integrato nei modelli senza un'adeguata sanificazione. Il processo prevede i seguenti passaggi: L'aggressore identifica innanzitutto le parti dell'applicazione in cui l'input dell'utente viene accettato e incluso direttamente nel modello. Ciò potrebbe avvenire nei moduli, nei parametri URL o in qualsiasi campo di input. Quindi, l'aggressore crea un payload che include codice dannoso progettato per sfruttare le capacità del motore di template per eseguire il codice. Successivamente, l'attaccante invia il payload predisposto attraverso i punti di input identificati. Poiché l'input non è stato disinfettato, il payload viene inserito direttamente nel modello. Quando il motore del modello elabora il modello, sostituisce i segnaposto con i dati forniti, incluso il payload dannoso. Se il motore del modello non è protetto, esegue il codice iniettato. Infine, il codice iniettato viene eseguito sul server, consentendo all'aggressore di eseguire azioni non autorizzate. Ciò potrebbe variare dal furto di dati all'esecuzione di comandi sul server, portando potenzialmente a una compromissione completa del sistema.
Motori di template comuni e relative vulnerabilità
Panoramica dei motori di template più diffusi
- Jinja2 (Python):
Jinja2 è un motore di template flessibile e potente utilizzato in molti framework web Python. Consente agli sviluppatori di creare contenuti web dinamici con facilità incorporando espressioni e logiche simili a Python direttamente nei modelli.
- Ramoscello (PHP):
Twig è un motore di template ampiamente utilizzato nelle applicazioni PHP. Offre una sintassi pulita e leggibile, che semplifica la scrittura e la manutenzione dei modelli per gli sviluppatori. Twig offre anche funzionalità come l'ereditarietà dei modelli e l'escape automatico, che aiutano a creare modelli sicuri e riutilizzabili.
- FreeMarker (Java):
FreeMarker è un motore di template basato su Java utilizzato in molte applicazioni aziendali. Supporta modelli di dati complessi e offre ampie funzionalità per la generazione di contenuti dinamici. FreeMarker viene spesso utilizzato in applicazioni su larga scala in cui la flessibilità e le prestazioni dei modelli sono fondamentali.
Impatti della SSTI
- Potenziali danni causati dagli attacchi SSTI
Le vulnerabilità SSTI possono portare a gravi conseguenze per le applicazioni web e i loro utenti:
- Esecuzione di codice in remoto: Gli aggressori sfruttano SSTI per eseguire codice arbitrario sul server. Ciò può portare alla completa compromissione del server, consentendo agli aggressori di assumere il controllo, installare malware o interrompere i servizi.
- Furto di dati: Le informazioni sensibili archiviate sul server, come dati personali, registri finanziari e informazioni aziendali proprietarie, possono essere accessibili e rubate dagli aggressori che sfruttano le vulnerabilità SSTI.
- Compromissione del sistema: SSTI può compromettere l'intera infrastruttura server, consentendo agli aggressori di aumentare i privilegi, ottenere un accesso persistente e passare ad altri sistemi all'interno della rete.
- Casi di studio ed esempi reali
Gli incidenti nel mondo reale evidenziano la gravità delle vulnerabilità SSTI:
- Caso di studio 1: Un sito di e-commerce ha subito un problema di sicurezza violazione a causa di SSTI, con conseguente furto dei dati dei clienti, comprese le informazioni di pagamento e i dati personali.
- Caso di studio 2: Un istituto finanziario ha subito un incidente di sicurezza in cui SSTI è stato sfruttato per accedere a registri riservati e dati finanziari sensibili dei clienti.
- Conseguenze per aziende e utenti
Le conseguenze dell'SSTI vanno oltre gli impatti tecnici e influiscono sulle operazioni aziendali e sulla fiducia degli utenti:
- Perdite finanziarie: Le organizzazioni devono affrontare danni finanziari significativi a causa di violazioni dei dati, tempi di inattività per la correzione e potenziali multe o sanzioni per la mancata conformità alle normative sulla protezione dei dati.
- Danni alla reputazione: Le violazioni dell'SSTI possono portare alla perdita della fiducia dei clienti, alla pubblicità negativa e al danneggiamento della reputazione dell'organizzazione sul mercato.
- Conseguenze legali: Le aziende potrebbero dover affrontare azioni legali, indagini normative e sanzioni per non aver protetto i dati degli utenti e non aver rispettato le leggi sulla protezione dei dati come GDPR, CCPA e altre.
Esempio tecnico
- Esempio di codice vulnerabile:
da flask import Flask, request, render_template_stringapp = Flask (__name__) @app .route ('/greet') def greet (): user_name = request.args.get ('name') template = f"Ciao, {user_name}!» restituisce render_template_string (template) se __name__ == '__main__': app.run ()
Spiegazione: In questo esempio, nome_utente
il parametro della richiesta viene inserito direttamente nella stringa del modello senza alcuna sanificazione. Un utente malintenzionato può sfruttarlo iniettando codice dannoso.
Esempio di sfruttamento: Accedendo all'URL http://localhost:5000/greet? nome= {{7*7}}
, un aggressore può iniettare {{7*7}}
nel modello, ottenendo l'output Ciao, 49!
. Ciò dimostra l'esecuzione del codice all'interno del modello.
- Esempio di codice mitigato:
da flask import Flask, request, render_template_stringimport jinja2app = Flask (__name__) @app .route ('/greet') def greet (): user_name = request.args.get ('name', 'Guest') template = «Ciao, {{user_name}}!» restituisce render_template_string (template, user_name=jinja2.escape (user_name)) se __name__ == '__main__': app.run ()
Spiegazione: Nell'esempio mitigato, jinja2.escape
viene utilizzato per disinfettare l'input dell'utente, assicurando che qualsiasi codice iniettato venga trattato come testo normale anziché come codice eseguibile
Conclusione
La Server-Side Template Injection (SSTI) rappresenta un rischio di sicurezza critico per le applicazioni Web, poiché consente agli aggressori di eseguire codice dannoso sfruttando la gestione degli input all'interno dei modelli lato server. Comprendere i meccanismi di SSTI, ad esempio il modo in cui i motori di template elaborano i dati forniti dagli utenti, è fondamentale per gli sviluppatori e i team di sicurezza.
La mitigazione delle vulnerabilità SSTI richiede l'adozione di pratiche di codifica sicure, come la convalida completa degli input e la corretta sanificazione degli input degli utenti prima che vengano integrati nei modelli. Ad esempio, l'utilizzo di funzioni come jinja2.escape nel motore Jinja2 di Python impedisce che gli input degli utenti vengano interpretati come codice eseguibile.
Gli incidenti nel mondo reale sottolineano le gravi conseguenze dell'SSTI, tra cui violazioni dei dati, interruzioni operative e danni alla reputazione. Questi incidenti evidenziano la necessità per le organizzazioni di dare priorità alle misure di sicurezza e rimanere vigili contro le minacce emergenti.
In conclusione, implementando sicurezza proattiva misurando e mantenendo la consapevolezza dei rischi SSTI, le organizzazioni possono proteggere efficacemente le proprie applicazioni web dallo sfruttamento e sostenere l'integrità dei propri sistemi e dati.
Pronti a vedere di persona?
Prova tu stesso tutte le funzionalità della piattaforma. Nessun impegno e nessuna carta di credito!

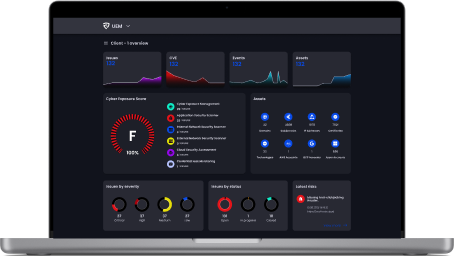